iOS中ImageIO框架详解与应用分析
一、引言
ImageIO框架提供了读取与写入图片数据的基本方法,使用它可以直接获取到图片文件的内容数据,ImageIO框架中包含6个头文件,其中完成主要功能的是前两个头文件中定义的方法:
1.CGImageSource.h:负责读取图片数据。
2.CGImageDestination.h:负责写入图片数据。
3.CGImageMetadata.h:图片文件元数据类。
4.CGImageProperties:定义了框架中使用的字符串常量和宏。
5.ImageIOBase.h:预处理逻辑,无需关心。
二、CGImageSource详解
CGImageSource类的主要作用是用来读取图片数据,在平时开发中,关于图片我们使用的最多的可能是UIImage类,UIImage是iOS系统UI系统中用于构建图像对象的类,但是其中只有图像数据,实际上一个图片文件中存储的除了图片数据外,还有一些地理位置、设备类型、时间等信息,除此之外,一个图片文件中可能存储的也不只一张图像(例如gif文件)。CGImageSource就是这样的一个抽象图片数据示例,从其中可以获取到我们所关心的所有数据。
读取图片文件数据,并将其展示在视图的简单代码示例如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| NSString * path = [[NSBundle mainBundle]pathForResource:@"timg" ofType:@"jpeg"]; NSURL * url = [NSURL fileURLWithPath:path]; CGImageRef myImage = NULL; CGImageSourceRef myImageSource;
myImageSource = CGImageSourceCreateWithURL((CFURLRef)url, NULL);
myImage = CGImageSourceCreateImageAtIndex(myImageSource, 0, NULL); CFRelease(myImageSource); UIImageView * image = [[UIImageView alloc]initWithFrame:CGRectMake(0, 0, 200, 200)]; image.image = [UIImage imageWithCGImage:myImage]; [self.view addSubview:image];
|
上面的示例代码采用的是本地的一个素材文件,当然通过网络图片链接也是可以创建CGImageSource独享的。除了通过URL链接的方式创建对象,ImageIO框架中还提供了两种方法,解析如下:
1 2 3 4 5 6 7
|
CGImageSourceRef __nullable CGImageSourceCreateWithDataProvider(CGDataProviderRef __nonnull provider, CFDictionaryRef __nullable options);
CGImageSourceRef __nullable CGImageSourceCreateWithData(CFDataRef __nonnull data, CFDictionaryRef __nullable options);
|
需要注意,上面所提到的所有创建CGImageSource的方法中都可以传入一个CFDictionaryRef类型的字典,可以配置的键值意义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
const CFStringRef kCGImageSourceTypeIdentifierHint;
const CFStringRef kCGImageSourceShouldCache;
const CFStringRef kCGImageSourceShouldAllowFloa;
const CFStringRef kCGImageSourceCreateThumbnailFromImageIfAbsent;
const CFStringRef kCGImageSourceCreateThumbnailFromImageAlways;
const CFStringRef kCGImageSourceThumbnailMaxPixelSize;
const CFStringRef kCGImageSourceCreateThumbnailWithTransform;
|
CGImageSource类中其他方法解析如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| CFTypeID CGImageSourceGetTypeID (void);
CFArrayRef __nonnull CGImageSourceCopyTypeIdentifiers(void);
CFStringRef __nullable CGImageSourceGetType(CGImageSourceRef __nonnull isrc);
size_t CGImageSourceGetCount(CGImageSourceRef __nonnull isrc);
CFDictionaryRef __nullable CGImageSourceCopyProperties(CGImageSourceRef __nonnull isrc, CFDictionaryRef __nullable options);
CFDictionaryRef __nullable CGImageSourceCopyPropertiesAtIndex(CGImageSourceRef __nonnull isrc, size_t index, CFDictionaryRef __nullable options);
CGImageMetadataRef __nullable CGImageSourceCopyMetadataAtIndex (CGImageSourceRef __nonnull isrc, size_t index, CFDictionaryRef __nullable options);
CGImageRef __nullable CGImageSourceCreateImageAtIndex(CGImageSourceRef __nonnull isrc, size_t index, CFDictionaryRef __nullable options);
void CGImageSourceRemoveCacheAtIndex(CGImageSourceRef __nonnull isrc, size_t index);
CGImageRef __nullable CGImageSourceCreateThumbnailAtIndex(CGImageSourceRef __nonnull isrc, size_t index, CFDictionaryRef __nullable options);
CGImageSourceRef __nonnull CGImageSourceCreateIncremental(CFDictionaryRef __nullable options);
void CGImageSourceUpdateData(CGImageSourceRef __nonnull isrc, CFDataRef __nonnull data, bool final);
void CGImageSourceUpdateDataProvider(CGImageSourceRef __nonnull isrc, CGDataProviderRef __nonnull provider, bool final);
CGImageSourceStatus CGImageSourceGetStatus(CGImageSourceRef __nonnull isrc);
CGImageSourceStatus CGImageSourceGetStatusAtIndex(CGImageSourceRef __nonnull isrc, size_t index);
|
三、CGImageDestination详解
CGImageSource是图片文件数据的抽象对象,而CGImageDestination的作用则是将抽象的图片数据写入指定的目标中。将图片写成文件示例如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| NSArray *paths=NSSearchPathForDirectoriesInDomains(NSDocumentDirectory,NSUserDomainMask,YES); NSString *newPath = [paths.firstObject stringByAppendingPathComponent:[NSString stringWithFormat:@"image.png"]]; CFURLRef URL = CFURLCreateWithFileSystemPath ( kCFAllocatorDefault, (CFStringRef)newPath, kCFURLPOSIXPathStyle, false);
CGImageDestinationRef myImageDest = CGImageDestinationCreateWithURL(URL,CFSTR("public.png"), 1, NULL); UIImage * image = [UIImage imageNamed:@"timg.jpeg"];
CGImageDestinationAddImage(myImageDest, image.CGImage, NULL); CGImageDestinationFinalize(myImageDest); CFRelease(myImageDest);
|
同样,除了可以直接将图片数据写入url外,也可以Data数据或数据消费器,方法如下:
1 2 3 4
| CGImageDestinationRef __nullable CGImageDestinationCreateWithDataConsumer(CGDataConsumerRef __nonnull consumer, CFStringRef __nonnull type, size_t count, CFDictionaryRef __nullable options);
CGImageDestinationRef __nullable CGImageDestinationCreateWithData(CFMutableDataRef __nonnull data, CFStringRef __nonnull type, size_t count, CFDictionaryRef __nullable options);
|
需要注意,上面方法的type参数设置写入数据的文件格式,必须为ImageIO框架所支持的格式,前面有方法可以获取所有支持的格式,还有一点,这3个写入方法的中options参数目前并没有什么作用,其是留给未来使用的,目前传入NULL即可。
CGImageDestination类中的其他方法解析如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| CFTypeID CGImageDestinationGetTypeID(void);
CFArrayRef __nonnull CGImageDestinationCopyTypeIdentifiers(void);
void CGImageDestinationSetProperties(CGImageDestinationRef __nonnull idst, CFDictionaryRef __nullable properties);
void CGImageDestinationAddImage(CGImageDestinationRef __nonnull idst, CGImageRef __nonnull image, CFDictionaryRef __nullable properties);
void CGImageDestinationAddImageFromSource(CGImageDestinationRef __nonnull idst, CGImageSourceRef __nonnull isrc, size_t index, CFDictionaryRef __nullable properties);
bool CGImageDestinationFinalize(CGImageDestinationRef __nonnull idst);
void CGImageDestinationAddImageAndMetadata(CGImageDestinationRef __nonnull idst, CGImageRef __nonnull image, CGImageMetadataRef __nullable metadata, CFDictionaryRef __nullable options);
bool CGImageDestinationCopyImageSource(CGImageDestinationRef __nonnull idst, CGImageSourceRef __nonnull isrc, CFDictionaryRef __nullable options, __nullable CFErrorRef * __nullable err);
|
上面列举的方法中,CGImageDestinationCopyImageSource()方法中的options参数可以添加一些图片的元信息,可以设置的键值对意义如下:
1 2 3 4 5 6 7 8 9 10 11 12
| const CFStringRef kCGImageDestinationMetadata;
const CFStringRef kCGImageDestinationMergeMetadata;
const CFStringRef kCGImageMetadataShouldExcludeXMP;
const CFStringRef kCGImageMetadataShouldExcludeGPS;
const CFStringRef kCGImageDestinationDateTime;
const CFStringRef kCGImageDestinationOrientation;
|
前面我们很多次提到元数据,CGImageMetadata类就是元数据的抽象,其中封装了一些方法供开发者读取或写入元数据信息。奇怪的是Apple的官方文档与API文档中并没有CGImageMetadata的介绍与解释,博客中本部分的内容,多出自我的理解,有疏漏和不对的地方,清楚的朋友可以指点与建议。
前边介绍,CGImageSource中有获取图片元数据的方法,CGImageDestination中也有写入图片元数据的方法,元数据中抽象出的CGImageMetadataTag是对具体数据内容的封装。CGImageMetadata解析如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| CFTypeID CGImageMetadataGetTypeID(void);
CGMutableImageMetadataRef __nonnull CGImageMetadataCreateMutable(void);
CGMutableImageMetadataRef __nullable CGImageMetadataCreateMutableCopy(CGImageMetadataRef __nonnull metadata);
CFTypeID CGImageMetadataTagGetTypeID(void);
CGImageMetadataTagRef __nullable CGImageMetadataTagCreate (CFStringRef __nonnull xmlns, CFStringRef __nullable prefix, CFStringRef __nonnull name, CGImageMetadataType type, CFTypeRef __nonnull value);
|
上面创建CGImageMetadataTag的方法中,xmlns设置命名空间,必须使用一个预定义的命名空间或者自定义的命名空间,对于自定义的命名空间,必须遵守Adobe的XMP规范。一些共用的命名空间定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| const CFStringRef kCGImageMetadataNamespaceExif;
const CFStringRef kCGImageMetadataNamespaceExifAux;
const CFStringRef kCGImageMetadataNamespaceExifEX;
const CFStringRef kCGImageMetadataNamespaceDublinCore;
const CFStringRef kCGImageMetadataNamespaceIPTCCore;
const CFStringRef kCGImageMetadataNamespacePhotoshop;
const CFStringRef kCGImageMetadataNamespaceTIFF;
const CFStringRef kCGImageMetadataNamespaceXMPBasic;
const CFStringRef kCGImageMetadataNamespaceXMPRights;
|
上面创建CGImageMetadataTag的方法中prefix设置命名空间缩写或前缀,同样一些公用的前缀定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| const CFStringRef kCGImageMetadataPrefixExif;
const CFStringRef kCGImageMetadataPrefixExifAux;
const CFStringRef kCGImageMetadataPrefixExifEX;
const CFStringRef kCGImageMetadataPrefixDublinCore;
const CFStringRef kCGImageMetadataPrefixIPTCCore;
const CFStringRef kCGImageMetadataPrefixPhotoshop;
const CFStringRef kCGImageMetadataPrefixTIFF;
const CFStringRef kCGImageMetadataPrefixXMPBasic;
const CFStringRef kCGImageMetadataPrefixXMPRights;
|
上面创建CGImageMetadataTag的方法中type设置对应值的类型,其是一个CGImageMetadataType类型的枚举,意义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| typedef CF_ENUM(int32_t, CGImageMetadataType) { kCGImageMetadataTypeInvalid = -1, kCGImageMetadataTypeDefault = 0, kCGImageMetadataTypeString = 1, kCGImageMetadataTypeArrayUnordered = 2, kCGImageMetadataTypeArrayOrdered = 3, kCGImageMetadataTypeAlternateArray = 4, kCGImageMetadataTypeAlternateText = 5, kCGImageMetadataTypeStructure = 6 };
|
获取到CGImageMetadataTag后,可以通过如下方法来获取其中封装的信息:
1 2 3 4 5 6 7 8 9 10 11 12
| CFStringRef __nullable CGImageMetadataTagCopyNamespace(CGImageMetadataTagRef __nonnull tag);
CFStringRef __nullable CGImageMetadataTagCopyPrefix(CGImageMetadataTagRef __nonnull tag);
CFStringRef __nullable CGImageMetadataTagCopyName(CGImageMetadataTagRef __nonnull tag);
CFTypeRef __nullable CGImageMetadataTagCopyValue(CGImageMetadataTagRef __nonnull tag);
CGImageMetadataType CGImageMetadataTagGetType(CGImageMetadataTagRef __nonnull tag);
CFArrayRef __nullable CGImageMetadataTagCopyQualifiers(CGImageMetadataTagRef __nonnull tag);
|
下面这些方法用于向CGImageMetadata中添加标签或者获取标签:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| CFArrayRef __nullable CGImageMetadataCopyTags(CGImageMetadataRef __nonnull metadata);
CGImageMetadataTagRef __nullable CGImageMetadataCopyTagWithPath(CGImageMetadataRef __nonnull metadata, CGImageMetadataTagRef __nullable parent, CFStringRef __nonnull path);
CFStringRef __nullable CGImageMetadataCopyStringValueWithPath(CGImageMetadataRef __nonnull metadata, CGImageMetadataTagRef __nullable parent, CFStringRef __nonnull path);
bool CGImageMetadataRegisterNamespaceForPrefix(CGMutableImageMetadataRef __nonnull metadata, CFStringRef __nonnull xmlns, CFStringRef __nonnull prefix, __nullable CFErrorRef * __nullable err);
bool CGImageMetadataSetTagWithPath(CGMutableImageMetadataRef __nonnull metadata, CGImageMetadataTagRef __nullable parent, CFStringRef __nonnull path, CGImageMetadataTagRef __nonnull tag);
bool CGImageMetadataSetValueWithPath(CGMutableImageMetadataRef __nonnull metadata, CGImageMetadataTagRef __nullable parent, CFStringRef __nonnull path, CFTypeRef __nonnull value);
bool CGImageMetadataRemoveTagWithPath(CGMutableImageMetadataRef __nonnull metadata, CGImageMetadataTagRef __nullable parent, CFStringRef __nonnull path);
void CGImageMetadataEnumerateTagsUsingBlock(CGImageMetadataRef __nonnull metadata, CFStringRef __nullable rootPath, CFDictionaryRef __nullable options, CGImageMetadataTagBlock __nonnull block);
|
五、CGImageProperties中定义的字典意义
前面提到的CGImageSourceCopyProperties方法与CGImageSourceCopyPropertiesAtIndex方法都会返回一个字典,字典中可能包含如下有意义的键:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| const CFStringRef kCGImagePropertyTIFFDictionary; /GIF信息字典 const CFStringRef kCGImagePropertyGIFDictionary;
const CFStringRef kCGImagePropertyJFIFDictionary;
const CFStringRef kCGImagePropertyExifDictionary;
const CFStringRef kCGImagePropertyPNGDictionary;
const CFStringRef kCGImagePropertyIPTCDictionary;
const CFStringRef kCGImagePropertyGPSDictionary;
const CFStringRef kCGImagePropertyRawDictionary;
const CFStringRef kCGImagePropertyCIFFDictionary;
const CFStringRef kCGImagePropertyMakerCanonDictionary;
const CFStringRef kCGImagePropertyMakerNikonDictionary;
const CFStringRef kCGImagePropertyMakerMinoltaDictionary;
const CFStringRef kCGImagePropertyMakerFujiDictionary;
const CFStringRef kCGImagePropertyMakerOlympusDictionary;
const CFStringRef kCGImagePropertyMakerPentaxDictionary;
const CFStringRef kCGImageProperty8BIMDictionary;
const CFStringRef kCGImagePropertyDNGDictionary ;
const CFStringRef kCGImagePropertyExifAuxDictionary;
const CFStringRef kCGImagePropertyOpenEXRDictionary;
const CFStringRef kCGImagePropertyMakerAppleDictionary ;
|
CGImageSourceCopyProperties方法返回的字典中还可能会有如下一个特殊的键:
1 2
| const CFStringRef kCGImagePropertyFileSize;
|
CGImageSourceCopyPropertiesAtIndex方法中可能包含的特殊键:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| const CFStringRef kCGImagePropertyPixelHeight;
const CFStringRef kCGImagePropertyPixelWidth;
const CFStringRef kCGImagePropertyDPIHeight;
const CFStringRef kCGImagePropertyDPIWidth;
const CFStringRef kCGImagePropertyDepth;
const CFStringRef kCGImagePropertyOrientation;
const CFStringRef kCGImagePropertyIsFloat;
const CFStringRef kCGImagePropertyIsIndexed;
const CFStringRef kCGImagePropertyHasAlpha;
const CFStringRef kCGImagePropertyColorModel;
const CFStringRef kCGImagePropertyProfileName;
|
kCGImagePropertyColorModel键可返回的值有如下几种定义:
1 2 3 4 5 6 7 8
| const CFStringRef kCGImagePropertyColorModelRGB;
const CFStringRef kCGImagePropertyColorModelGray;
const CFStringRef kCGImagePropertyColorModelCMYK;
const CFStringRef kCGImagePropertyColorModelLab;
|
kCGImagePropertyTIFFDictionary键可返回的值定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| const CFStringRef kCGImagePropertyTIFFCompression;
const CFStringRef kCGImagePropertyTIFFPhotometricInterpretation;
const CFStringRef kCGImagePropertyTIFFDocumentName;
const CFStringRef kCGImagePropertyTIFFImageDescription;
const CFStringRef kCGImagePropertyTIFFMake;
const CFStringRef kCGImagePropertyTIFFModel;
const CFStringRef kCGImagePropertyTIFFOrientation;
const CFStringRef kCGImagePropertyTIFFXResolution;
const CFStringRef kCGImagePropertyTIFFYResolution;
const CFStringRef kCGImagePropertyTIFFResolutionUnit;
const CFStringRef kCGImagePropertyTIFFSoftware;
const CFStringRef kCGImagePropertyTIFFTransferFunction;
const CFStringRef kCGImagePropertyTIFFDateTime;
const CFStringRef kCGImagePropertyTIFFArtist;
const CFStringRef kCGImagePropertyTIFFHostComputer;
const CFStringRef kCGImagePropertyTIFFCopyright;
const CFStringRef kCGImagePropertyTIFFWhitePoint;
const CFStringRef kCGImagePropertyTIFFPrimaryChromaticities;
const CFStringRef kCGImagePropertyTIFFTileWidth;
const CFStringRef kCGImagePropertyTIFFTileLength;
|
kCGImagePropertyJFIFDictionary对应的字典中可能包含如下意义的键:
1 2 3 4 5 6 7 8 9 10
| const CFStringRef kCGImagePropertyJFIFVersion;
const CFStringRef kCGImagePropertyJFIFXDensity;
const CFStringRef kCGImagePropertyJFIFYDensity;
const CFStringRef kCGImagePropertyJFIFDensityUnit;
const CFStringRef kCGImagePropertyJFIFIsProgressive;
|
kCGImagePropertyExifDictionary对应的字典中可能包含如下意义的键 :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134
| const CFStringRef kCGImagePropertyExifExposureTime;
const CFStringRef kCGImagePropertyExifFNumber;
const CFStringRef kCGImagePropertyExifExposureProgram;
const CFStringRef kCGImagePropertyExifSpectralSensitivity;
const CFStringRef kCGImagePropertyExifISOSpeedRatings;
const CFStringRef kCGImagePropertyExifOECF;
const CFStringRef kCGImagePropertyExifSensitivityType;
const CFStringRef kCGImagePropertyExifStandardOutputSensitivity;
const CFStringRef kCGImagePropertyExifRecommendedExposureIndex;
const CFStringRef kCGImagePropertyExifISOSpeed; const CFStringRef kCGImagePropertyExifISOSpeedLatitudeyyy; const CFStringRef kCGImagePropertyExifISOSpeedLatitudezzz;
const CFStringRef kCGImagePropertyExifVersion;
const CFStringRef kCGImagePropertyExifDateTimeOriginal;
const CFStringRef kCGImagePropertyExifDateTimeDigitized;
const CFStringRef kCGImagePropertyExifComponentsConfiguration;
const CFStringRef kCGImagePropertyExifCompressedBitsPerPixel;
const CFStringRef kCGImagePropertyExifShutterSpeedValue;
const CFStringRef kCGImagePropertyExifApertureValue;
const CFStringRef kCGImagePropertyExifBrightnessValue;
const CFStringRef kCGImagePropertyExifExposureBiasValue;
const CFStringRef kCGImagePropertyExifMaxApertureValue;
const CFStringRef kCGImagePropertyExifSubjectDistance;
const CFStringRef kCGImagePropertyExifMeteringMode;
const CFStringRef kCGImagePropertyExifLightSource;
const CFStringRef kCGImagePropertyExifFlash;
const CFStringRef kCGImagePropertyExifFocalLength;
const CFStringRef kCGImagePropertyExifSubjectArea;
const CFStringRef kCGImagePropertyExifMakerNote;
const CFStringRef kCGImagePropertyExifUserComment;
const CFStringRef kCGImagePropertyExifSubsecTime;
const CFStringRef kCGImagePropertyExifSubsecTimeOriginal;
const CFStringRef kCGImagePropertyExifSubsecTimeDigitized;
const CFStringRef kCGImagePropertyExifFlashPixVersion;
const CFStringRef kCGImagePropertyExifColorSpace;
const CFStringRef kCGImagePropertyExifPixelXDimension;
const CFStringRef kCGImagePropertyExifPixelYDimension;
const CFStringRef kCGImagePropertyExifRelatedSoundFile;
const CFStringRef kCGImagePropertyExifFlashEnergy;
const CFStringRef kCGImagePropertyExifSpatialFrequencyResponse;
const CFStringRef kCGImagePropertyExifFocalPlaneXResolution; const CFStringRef kCGImagePropertyExifFocalPlaneYResolution; const CFStringRef kCGImagePropertyExifFocalPlaneResolutionUnit;
const CFStringRef kCGImagePropertyExifSubjectLocation;
const CFStringRef kCGImagePropertyExifExposureIndex;
const CFStringRef kCGImagePropertyExifSensingMethod;
const CFStringRef kCGImagePropertyExifFileSource;
const CFStringRef kCGImagePropertyExifSceneType;
const CFStringRef kCGImagePropertyExifCFAPattern;
const CFStringRef kCGImagePropertyExifCustomRendered;
const CFStringRef kCGImagePropertyExifExposureMode;
const CFStringRef kCGImagePropertyExifWhiteBalance;
const CFStringRef kCGImagePropertyExifDigitalZoomRatio;
const CFStringRef kCGImagePropertyExifFocalLenIn35mmFilm;
const CFStringRef kCGImagePropertyExifSceneCaptureType;
const CFStringRef kCGImagePropertyExifGainControl;
const CFStringRef kCGImagePropertyExifContrast;
const CFStringRef kCGImagePropertyExifSaturation;
const CFStringRef kCGImagePropertyExifSharpness;
const CFStringRef kCGImagePropertyExifDeviceSettingDescription;
const CFStringRef kCGImagePropertyExifSubjectDistRange;
const CFStringRef kCGImagePropertyExifImageUniqueID;
const CFStringRef kCGImagePropertyExifCameraOwnerName;
const CFStringRef kCGImagePropertyExifBodySerialNumber;
const CFStringRef kCGImagePropertyExifLensSpecification;
const CFStringRef kCGImagePropertyExifLensMake;
const CFStringRef kCGImagePropertyExifLensModel;
const CFStringRef kCGImagePropertyExifLensSerialNumber;
const CFStringRef kCGImagePropertyExifGamma;
|
kCGImagePropertyExifAuxDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| const CFStringRef kCGImagePropertyExifAuxLensInfo;
const CFStringRef kCGImagePropertyExifAuxLensModel;
const CFStringRef kCGImagePropertyExifAuxSerialNumber;
const CFStringRef kCGImagePropertyExifAuxLensID;
const CFStringRef kCGImagePropertyExifAuxLensSerialNumber;
const CFStringRef kCGImagePropertyExifAuxImageNumber;
const CFStringRef kCGImagePropertyExifAuxFlashCompensation;
const CFStringRef kCGImagePropertyExifAuxOwnerName;
const CFStringRef kCGImagePropertyExifAuxFirmware;
|
kCGImagePropertyGIFDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9
| const CFStringRef kCGImagePropertyGIFLoopCount;
const CFStringRef kCGImagePropertyGIFDelayTime;
const CFStringRef kCGImagePropertyGIFImageColorMap; const CFStringRef kCGImagePropertyGIFHasGlobalColorMap;
const CFStringRef kCGImagePropertyGIFUnclampedDelayTime;
|
kCGImagePropertyPNGDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| const CFStringRef kCGImagePropertyPNGGamma;
const CFStringRef kCGImagePropertyPNGInterlaceType;
const CFStringRef kCGImagePropertyPNGXPixelsPerMeter;
const CFStringRef kCGImagePropertyPNGYPixelsPerMeter;
const CFStringRef kCGImagePropertyPNGsRGBIntent;
const CFStringRef kCGImagePropertyPNGChromaticities;
const CFStringRef kCGImagePropertyPNGAuthor;
const CFStringRef kCGImagePropertyPNGCopyright;
const CFStringRef kCGImagePropertyPNGCreationTime;
const CFStringRef kCGImagePropertyPNGDescription;
const CFStringRef kCGImagePropertyPNGModificationTime;
const CFStringRef kCGImagePropertyPNGSoftware;
const CFStringRef kCGImagePropertyPNGTitle;
const CFStringRef kCGImagePropertyAPNGLoopCount;
const CFStringRef kCGImagePropertyAPNGDelayTime; const CFStringRef kCGImagePropertyAPNGUnclampedDelayTime;
|
kCGImagePropertyGPSDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| const CFStringRef kCGImagePropertyGPSVersion;
const CFStringRef kCGImagePropertyGPSLatitudeRef;
const CFStringRef kCGImagePropertyGPSLatitude;
const CFStringRef kCGImagePropertyGPSLongitudeRef;
const CFStringRef kCGImagePropertyGPSLongitude;
const CFStringRef kCGImagePropertyGPSAltitudeRef;
const CFStringRef kCGImagePropertyGPSAltitude;
const CFStringRef kCGImagePropertyGPSTimeStamp;
const CFStringRef kCGImagePropertyGPSSatellites;
const CFStringRef kCGImagePropertyGPSStatus;
const CFStringRef kCGImagePropertyGPSMeasureMode;
const CFStringRef kCGImagePropertyGPSDOP;
const CFStringRef kCGImagePropertyGPSSpeedRef;
const CFStringRef kCGImagePropertyGPSSpeed;
const CFStringRef kCGImagePropertyGPSTrackRef;
const CFStringRef kCGImagePropertyGPSTrack;
const CFStringRef kCGImagePropertyGPSImgDirectionRef;
const CFStringRef kCGImagePropertyGPSImgDirection;
const CFStringRef kCGImagePropertyGPSMapDatum;
const CFStringRef kCGImagePropertyGPSDestLatitudeRef;
const CFStringRef kCGImagePropertyGPSDestLatitude;
const CFStringRef kCGImagePropertyGPSDestLongitudeRef;
const CFStringRef kCGImagePropertyGPSDestLongitude;
const CFStringRef kCGImagePropertyGPSDestBearingRef;
const CFStringRef kCGImagePropertyGPSDestBearing;
const CFStringRef kCGImagePropertyGPSDestDistanceRef;
const CFStringRef kCGImagePropertyGPSDestDistance;
const CFStringRef kCGImagePropertyGPSProcessingMethod;
const CFStringRef kCGImagePropertyGPSAreaInformation;
const CFStringRef kCGImagePropertyGPSDateStamp;
const CFStringRef kCGImagePropertyGPSDifferental;
const CFStringRef kCGImagePropertyGPSHPositioningError;
|
kCGImagePropertyIPTCDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| const CFStringRef kCGImagePropertyIPTCObjectTypeReference;
const CFStringRef kCGImagePropertyIPTCObjectAttributeReference;
const CFStringRef kCGImagePropertyIPTCObjectName;
const CFStringRef kCGImagePropertyIPTCEditStatus;
const CFStringRef kCGImagePropertyIPTCEditorialUpdate;
const CFStringRef kCGImagePropertyIPTCUrgency;
const CFStringRef kCGImagePropertyIPTCSubjectReference;
const CFStringRef kCGImagePropertyIPTCCategory;
const CFStringRef kCGImagePropertyIPTCSupplementalCategory;
const CFStringRef kCGImagePropertyIPTCFixtureIdentifier;
const CFStringRef kCGImagePropertyIPTCKeywords;
const CFStringRef kCGImagePropertyIPTCContentLocationCode;
const CFStringRef kCGImagePropertyIPTCContentLocationName;
const CFStringRef kCGImagePropertyIPTCReleaseDate;
const CFStringRef kCGImagePropertyIPTCReleaseTime;
const CFStringRef kCGImagePropertyIPTCExpirationDate;
const CFStringRef kCGImagePropertyIPTCExpirationTime;
const CFStringRef kCGImagePropertyIPTCSpecialInstructions;
const CFStringRef kCGImagePropertyIPTCActionAdvised;
const CFStringRef kCGImagePropertyIPTCReferenceService;
const CFStringRef kCGImagePropertyIPTCReferenceDate;
const CFStringRef kCGImagePropertyIPTCReferenceNumber;
const CFStringRef kCGImagePropertyIPTCDateCreated;
const CFStringRef kCGImagePropertyIPTCTimeCreated;
const CFStringRef kCGImagePropertyIPTCDigitalCreationDate;
const CFStringRef kCGImagePropertyIPTCDigitalCreationTime;
const CFStringRef kCGImagePropertyIPTCOriginatingProgram;
const CFStringRef kCGImagePropertyIPTCProgramVersion; 图像的编辑周期(早晨,晚上或两者)。 const CFStringRef kCGImagePropertyIPTCObjectCycle;
const CFStringRef kCGImagePropertyIPTCByline;
const CFStringRef kCGImagePropertyIPTCBylineTitle;
const CFStringRef kCGImagePropertyIPTCCity;
const CFStringRef kCGImagePropertyIPTCSubLocation;
const CFStringRef kCGImagePropertyIPTCProvinceState;
const CFStringRef kCGImagePropertyIPTCCountryPrimaryLocationCode;
const CFStringRef kCGImagePropertyIPTCCountryPrimaryLocationName;
const CFStringRef kCGImagePropertyIPTCOriginalTransmissionReference;
const CFStringRef kCGImagePropertyIPTCHeadline;
const CFStringRef kCGImagePropertyIPTCCredit;
const CFStringRef kCGImagePropertyIPTCSource;
const CFStringRef kCGImagePropertyIPTCCopyrightNotice;
const CFStringRef kCGImagePropertyIPTCContact;
const CFStringRef kCGImagePropertyIPTCCaptionAbstract;
const CFStringRef kCGImagePropertyIPTCWriterEditor;
const CFStringRef kCGImagePropertyIPTCImageType;
const CFStringRef kCGImagePropertyIPTCImageOrientation;
const CFStringRef kCGImagePropertyIPTCLanguageIdentifier;
const CFStringRef kCGImagePropertyIPTCStarRating;
const CFStringRef kCGImagePropertyIPTCCreatorContactInfo;
const CFStringRef kCGImagePropertyIPTCRightsUsageTerms;
const CFStringRef kCGImagePropertyIPTCScene;
|
上面的kCGImagePropertyIPTCCreatorContactInfo对应的字典中键的定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| const CFStringRef kCGImagePropertyIPTCContactInfoCity;
const CFStringRef kCGImagePropertyIPTCContactInfoCountry;
const CFStringRef kCGImagePropertyIPTCContactInfoAddress;
const CFStringRef kCGImagePropertyIPTCContactInfoPostalCode;
const CFStringRef kCGImagePropertyIPTCContactInfoStateProvince;
const CFStringRef kCGImagePropertyIPTCContactInfoEmails;
const CFStringRef kCGImagePropertyIPTCContactInfoPhones;
const CFStringRef kCGImagePropertyIPTCContactInfoWebURLs;
|
kCGImageProperty8BIMDictionary对应的字典中可能包含的键定义如下:
1 2 3 4
| const CFStringRef kCGImageProperty8BIMLayerNames;
const CFStringRef kCGImageProperty8BIMVersion;
|
kCGImagePropertyDNGDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| const CFStringRef kCGImagePropertyDNGVersion;
const CFStringRef kCGImagePropertyDNGBackwardVersion;
const CFStringRef kCGImagePropertyDNGUniqueCameraModel; const CFStringRef kCGImagePropertyDNGLocalizedCameraModel;
const CFStringRef kCGImagePropertyDNGCameraSerialNumber;
const CFStringRef kCGImagePropertyDNGLensInfo;
const CFStringRef kCGImagePropertyDNGBlackLevel;
const CFStringRef kCGImagePropertyDNGWhiteLevel;
const CFStringRef kCGImagePropertyDNGCalibrationIlluminant1; const CFStringRef kCGImagePropertyDNGCalibrationIlluminant2; const CFStringRef kCGImagePropertyDNGColorMatrix1; const CFStringRef kCGImagePropertyDNGColorMatrix2; const CFStringRef kCGImagePropertyDNGCameraCalibration1; const CFStringRef kCGImagePropertyDNGCameraCalibration2; const CFStringRef kCGImagePropertyDNGAsShotNeutral; const CFStringRef kCGImagePropertyDNGAsShotWhiteXY; const CFStringRef kCGImagePropertyDNGBaselineExposure; const CFStringRef kCGImagePropertyDNGBaselineNoise; const CFStringRef kCGImagePropertyDNGBaselineSharpness; const CFStringRef kCGImagePropertyDNGPrivateData; const CFStringRef kCGImagePropertyDNGCameraCalibrationSignature; const CFStringRef kCGImagePropertyDNGProfileCalibrationSignature; const CFStringRef kCGImagePropertyDNGNoiseProfile; const CFStringRef kCGImagePropertyDNGWarpRectilinear; const CFStringRef kCGImagePropertyDNGWarpFisheye; const CFStringRef kCGImagePropertyDNGFixVignetteRadial;
|
kCGImagePropertyCIFFDictionary对应的字典中可能包含的键定义如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| const CFStringRef kCGImagePropertyCIFFDescription;
const CFStringRef kCGImagePropertyCIFFFirmware;
const CFStringRef kCGImagePropertyCIFFOwnerName;
const CFStringRef kCGImagePropertyCIFFImageName;
const CFStringRef kCGImagePropertyCIFFImageFileName;
const CFStringRef kCGImagePropertyCIFFReleaseMethod;
const CFStringRef kCGImagePropertyCIFFReleaseTiming;
const CFStringRef kCGImagePropertyCIFFRecordID;
const CFStringRef kCGImagePropertyCIFFSelfTimingTime;
const CFStringRef kCGImagePropertyCIFFCameraSerialNumber;
const CFStringRef kCGImagePropertyCIFFImageSerialNumber;
const CFStringRef kCGImagePropertyCIFFContinuousDrive);
const CFStringRef kCGImagePropertyCIFFFocusMode;
const CFStringRef kCGImagePropertyCIFFMeteringMode;
const CFStringRef kCGImagePropertyCIFFShootingMode;
const CFStringRef kCGImagePropertyCIFFLensModel;
const CFStringRef kCGImagePropertyCIFFLensMaxMM;
const CFStringRef kCGImagePropertyCIFFLensMinMM;
const CFStringRef kCGImagePropertyCIFFWhiteBalanceIndex;
const CFStringRef kCGImagePropertyCIFFFlashExposureComp;
const CFStringRef kCGImagePropertyCIFFMeasuredEV);
|
六、ImageIO框架在实际开发中的几个应用
1.显示特殊格式的图片
在平时开发中,我们通常使用UIImage来读取图片,UIImage支持的图片包括png与jpg等,但是类似windows系统的ico图标,UIImage默认是无法显示的,可以通过ImageIO框架来在iOS系统中使用ico图标,示例如下:
1 2 3 4 5 6 7 8 9 10 11 12
| NSString * path = [[NSBundle mainBundle]pathForResource:@"image" ofType:@"ico"]; NSURL * url = [NSURL fileURLWithPath:path]; CGImageRef myImage = NULL; CGImageSourceRef myImageSource; CFDictionaryRef myOptions = NULL; myImageSource = CGImageSourceCreateWithURL((CFURLRef)url, NULL); myImage = CGImageSourceCreateImageAtIndex(myImageSource, 0, NULL); CFRelease(myImageSource); UIImageView * image = [[UIImageView alloc]initWithFrame:CGRectMake(0, 0, 200, 200)]; image.image = [UIImage imageWithCGImage:myImage];
|
2.读取数码相机拍摄图片的地理位置、时间等信息
3.对相册中图片的地理位置,时间等信息进行自定义修改。
4.将自定义格式的图片数据写入本地文件。
5.展示GIF动图
详情见博客:[https://my.oschina.net/u/2340880/blog/608560](https://my.oschina.net/u/2340880/blog/608560)。
6.渐进渲染大图
渐进渲染技术在对加载大图片时特别重要,你应该使用过地图软件,地图视图在加载时是局部进行加载,当移动或者放大时,地图会一部分一部分的渐进进行加载,使用ImageIO框架可以实现大图渐进渲染的效果,一般在对大图片进行网络请求时,可以获取一部分数据就加载一部分数据,为了便于演示,博客中使用定时器来默认网络返回数据,代码示例如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| @interface ViewController () { NSMutableData * _data; NSData * _allData; NSUInteger length; UIImageView * _imageView; NSTimer * timer; NSInteger le; } @end @implementation ViewController
- (void)viewDidLoad { [super viewDidLoad]; _data = [[NSMutableData alloc]init]; NSString * path = [[NSBundle mainBundle]pathForResource:@"Default-Portrait-ns@2x" ofType:@"png"]; _allData = [NSData dataWithContentsOfFile:path]; length = _allData.length; le = length/10; timer = [NSTimer scheduledTimerWithTimeInterval:1 target:self selector:@selector(updateImage) userInfo:nil repeats:YES]; _imageView = [[UIImageView alloc]initWithFrame:self.view.frame]; [self.view addSubview:_imageView]; }
-(void)updateImage{ static int index = 0; if (index==10) { return; } NSUInteger l; if (index==9) { l=length-le*9; }else{ l= le; } Byte by[l]; [_allData getBytes:by range:NSMakeRange(index*le, l)]; [_data appendBytes:by length:l]; CGImageSourceRef myImageSource = CGImageSourceCreateWithData((CFDataRef)_data, NULL); CGImageRef myImage = CGImageSourceCreateImageAtIndex(myImageSource, 0, NULL); CFRelease(myImageSource); _imageView.image = [UIImage imageWithCGImage:myImage]; index++; } @end
|
效果如下:
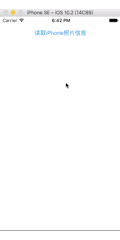